Data scientists may keep utilizing the well-known tools they are accustomed to using since MongoDB integrates seamlessly with the most popular data science tools and programming languages, including Python, R, etc. In this article, we are providing data fundamentals for Python and MongoDB. Discover what is the hierarchy of needs in data science.
Why Python and MongoDB for Data Science Processing?
Simply put, Python developers may leverage MongoDB to construct online applications, analyze data, or work on operations with tremendous success. This is because MongoDB stores data in a format like JSON, and Python stores data in a dictionary format.
Data is stored in MongoDB in adaptable, schema-free documents that resemble JSON. Rich libraries for Python allow you to process JSON and BSON data types directly.
Python and MongoDB work nicely together for data science processing through drivers like PyMongo, MongoEngine, and others. Because of this, MongoDB works well with Python by removing rigidity from the database schema.
What is data science?
Using machine learning and statistical methods to analyze unprocessed data and make inferences about it is known as data science. Thus, data science encompasses computer science, mathematics, statistics, and other fields. Learn more about what is data science.
The fact that Python is the most widely used and easiest-to-learn programming language among data science professionals surprises a lot of learners. MongoDB, a well-liked NoSQL database, can handle and store substantial amounts of semi-structured and unstructured data. Projects in data science that need to be flexible, scalable, and performant frequently employ it.
Suggested Read: Top 7 Data Science Applications and Real-Life Examples You Should Know
Python is one of the most popular programming languages for data science for many additional reasons, such as
Availability: A sizable number of reusable packages created by other users are readily available.
Speed: Python is comparatively faster than other programming languages in terms of speed.
Design objective: Python’s simple and intuitive syntax responsibilities make it easier to build apps with a readable codebase.
MongoDB is good for data science processing for the following reasons:
Schemas: They are versatile because MongoDB stores data in JSON objects. An entity may contain long, intricately nested fields, and distinct fields may be recorded for distinct object instances.
Speed: Compared to relational databases, indexing in this document structure often offers much faster data access.
Scalability: MongoDB divides the database into shards to efficiently manage large amounts of data.
Check your skills with our data science interview questions and answers.
Data Science Fundamentals for Python
As we’ve already mentioned, Python is popular among developers since it’s simple to learn and read. Python is becoming more and more popular and in demand because of its large library and framework collection, which includes NumPy, Pandas, Matplotlib, Seaborn, sci-kit-learn, TensorFlow, and PyTorch. Here are the data science fundamentals for Python. Explore why you should learn Python here.
Four key subjects make up a basic Python curriculum, and they are as follows:
- Data types such as int, float, and strings
- Compound data structures, including dictionaries, tuples, and lists
- Loops, functions, and conditionals
- Using external libraries with object-oriented programming
Let’s review each one and explore the essentials you need to know.
Understanding how Python interprets data is the first step.
Data types such as int, float, and strings
You should be familiar with integers (int), floats (float), strings (str), and booleans (bool), as these are the most commonly used data types.
Type, typecasting, and I/O functions
- Use the type() method to determine the type of data.
type(12)
#output: int
- Putting values into input-output functions and variables (a = 3.14)
- Converting a specific type of variable or data, if possible, into another type is known as typecasting. To transform a string of integers into an integer, for instance,
astring = “55”
print(type(astring))
# output: <class ‘str’>
astring = int(astring)
print(type(astring))
# output: <class ‘int64’>
- However, an error will be thrown if you attempt to convert an alphabetic or alphanumeric text to an integer:
astring = “3DRY”
print(type(astring))
class<’str’>
anum = int(astring)
print(anum)
#Output
ValueError Traceback (most recent call last)
<ipython-input-36-9cb57c73e9c> in <module>
—–> 1 anum = int(astring)
2 print(anum)
ValueError: invalid literal for int() with base 10: ‘3DRY’
After you understand how to use the fundamental data types, you should learn arithmetic operators, expression evaluations (DMAS), and storing the outcome in a variable for later use.
answer = 21 + 43 / 17 – 6 * 2
print(answer)
# output: 11.529411764705884
Strings
Working with the string data type requires some familiarity with textual data and its operators. Put these ideas into practice:
- Using the split() and join() methods to split and join the string, then concatenating the strings
- By utilizing the lower() and upper() methods, the string’s case can be changed.
- Utilizing a string’s substrings.
Recommended Read: Working with Data in Python: Data Cleaning, Wrangling, and Preprocessing.
Compound Data Structures
Lists and Tuples
Lists are among the most crucial and widely used data structures in Python. A list is made up of elements, which may or may not be of the same data type.
Knowing lists will ultimately enable you to calculate statistical models and algebraic equations using your variety of data. The following are the ideas you should understand:
- How Python list may hold several kinds of data.
- To access a particular element or sub-list within the list, use indexing and slicing.
- Helper methods for item deletion, reversing, copying, adding, and sorting.
- Lists inside lists are called nested lists. Take [1, 2, 3, [10, 11]] as an example.
- Addition to a list.
alist + alist
# output: [‘sla’, 2, 5.5, 10, [1, 2, 3], ‘sla’, 2, 5.5, 10, [1, 2, 3]]
alist * 2
# output: [‘sla’, 2, 5.5, 10, [1, 2, 3], ‘sla’, 2, 5.5, 10, [1, 2, 3]]
Tuples
An unchangeable, ordered sequence of objects is called a tuple. Although they are comparable to lists, the main distinction is that whereas lists are mutable, tuples are not.
Ideas to concentrate on:
- Slicing and indexing (like lists).
- tuples nested.
- tuples and auxiliary functions like index() and count() are added.
Dictionaries
In Python, they are an additional kind of collection. Dictionary entries are more akin to addresses than lists, which are integer-indexed. Dictionary entries consist of key-value pairs, where keys are comparable to list indexes.
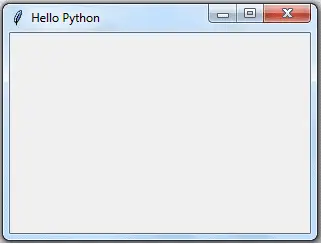
You must pass the key included in square brackets to access an element.
# dictionary
country_code = {‘India’ : 1, ‘USA’ : 2, ‘China’ : 3}
print(country_code)
{‘India’: 1, ‘USA’: 2, ‘China’: 3}
print(country_code[‘China’])
3
Ideas to concentrate on
- Iterating via a dictionary that has loops in it as well.
- Using utility functions such as update(), pop(), items(), get(), and so forth.
Learn to build web applications with Django, a Python web framework.
Conditionals, loops, and functions
To evaluate conditions, Python makes use of these boolean variables. Boolean values represent the outcome of any comparison or evaluation.
Conditions and branching
x = True
print(type(x))
# output: <class bool>
print(1 ==2)
# output: False
It’s important to pay close attention to the comparison in the graphic since some individuals mistake the comparison operator (==) for the assignment operator (=).
Boolean operators (or, and, not)
Together, these are utilized to assess complex claims.
- or – For the complete condition to hold true, at least one of the numerous comparisons must be accurate.
- and – For the entire condition to be true, each comparison must be true.
- not – Verifies the opposite of the given comparison.
a = True
not(a)
False
score = 76
percentile = 83
if score > 75 or percentile > 90:
print(“Admission successful!”)
else:
print(“Try again next year”)
# output: Try again next year
Ideas to acquire:
- To create your condition, use the if, else, and elif phrases.
- executing intricate comparisons in a single setting.
- Indentation is an important consideration when writing nested if/else expressions.
- employing the operators not, in, is, and boolean.
Loops
Loops will be your best friend in reducing the overhead of code redundancy when performing repeating tasks. Loops are useful when you need to run through every entry in a list or dictionary. There are two kinds of loops: while and for.
Pay attention to
- The range() function and for loop iteration across a list.
- while loops
age = [12,43,45,10]
i = 0
while i < len(age):
if age[i] >= 18:
print(“Adult”)
else:
print(“Juvenile”)
i += 1
# output:
# Juvenile
# Adult
# Adult
# Juvenile
- iterating through lists and adding elements in a specific order, or performing any other job involving list items
cubes = []
for i in range(1,10):
cubes.append(i ** 3)
print(cubes)
#output: [1, 8, 27, 64, 125, 216, 343, 512, 729]
- use the terms continue, pass, and break.
A complex and succinct approach of building a list using an iterable followed by a for clause.
For instance, you may use list comprehension to make the list of nine cubes that are displayed in the previous example.
cubes = [n** 3 for n in range(1,10)]
print(cubes)
# output: [1, 8, 27, 64, 125, 216, 343, 512, 729]
Functions
A function is a section of code that modifies input data and produces the intended result. Code that uses functions is easier to read, has less duplication, can be reused, and saves time.
Python creates code blocks by indenting them. Here’s an illustration of a function:
def add_two_numbers(a, b):
sum = a + b
return sum
The def keyword is used to define a function, which is then followed by the function’s name, its arguments (input), parentheses, and a colon.
The indented code block that makes up the function’s body returns the output when the return keyword is used.
According to its definition, you call a function by giving it its name and sending its arguments inside parentheses.
add_two_numbers(5, 11)
16
Object-Oriented Programming using External Libraries
In reality, we are interacting with a list class object or a ‘dict’ class object when we say list or ‘dict’. You may determine that a dictionary object is a class ‘dict’ object by printing its type.
adict = {‘US’ : 1897897}
print(type(adict))
<class ‘dict’>
These are all Python pre-defined classes that greatly simplify and expedite our tasks. Encapsulating variables (data) and functions into a single unit, objects are instances of a class. They can use the methods (functions) and variables (attributes) found in classes.
The following is the definition of a class and its object:
class Rectangle:
def __init__(self, height, width):
self.height = height
self.width = width
def area(self):
area = self.height * self.width
return area
rect1 = Rectangle(12, 10)
print(type(rect1))
# output: <class ‘__main__.Rectangle’>
After that, you can use the dot(.) operator to access the methods and attributes.
rect1.height
12
rect1.width
10
rect1.area()
120
External Libraries
Working on Python projects requires utilizing external libraries and modules. To help us with our work, these libraries and modules provide defined classes, properties, and methods. To perform our computations, for instance, the math library offers a variety of mathematical functions. ‘.py’ files make up the libraries. Explore the popular Python libraries for data analysis.
You should become proficient in:
- Import libraries in your system
import math
print(type(math))
<class ‘module’>
- Use the help feature to find out more about a function or library
- Directly import the necessary function.
from math import log, pi
from numpy import asarray
log(100, 100)
#output
2.0
Data Science Fundamentals for MongoDB
Database is the first word that springs to mind when discussing structured data. There are several kinds of databases; in this case, we’ll focus on NoSQL databases.
NoSQL databases have been the most widely used data storage solution over the past few years. Relational databases store data in a tabular style, whereas NoSQL databases, sometimes known as “non-SQL” or “not only SQL,” store data in a non-tabular format.
We will be working with MongoDB, a popular NoSQL database platform, and learning how to use data from MongoDB databases for data science today.
Check whether you have the following applications installed in your system,
- MongoDB
- MongoDB Compass
- Python 3.7 or above
- ‘pymongo’ module. It can be installed using ’pip install pymongo’
- Pandas for DataFrame creation. You can use any data science library as per your use case.
Suggested Article: RDBMS vs. NoSQL
Basics of MongoDB
MongoDB is a multipurpose document database made for cloud computing and contemporary application development. Because of its scale-out design, you may add more nodes to your system to share the load and meet the growing demand for it.
These are some of the most important ideas and words you will come across when studying MongoDB.
Records in a Document Database: Documents
The data is stored in MongoDB as JSON documents. The document data model is easy for developers to learn and use since it readily correlates to objects in application code.
A JSON document’s fields can change from one document to the next. In contrast, adding a field to a conventional relational database table adds a column to the database table and, by extension, to every record in the database.
Documents can store structures like arrays and express hierarchical relationships by being nested.
{
“model”: “Volvo c70”,
“year”: 2007,
“bodyStyle”: [“couple”, “convertible”],
“engine”:
{
“model”: “D5”,
“power”: “178hp”
}
}
With the document model, working with complex, dynamic, and chaotic data from several sources is made flexible. It makes it possible for developers to release new features for applications quickly.
To handle more data types and expedite internal access, MongoDB converts documents into a format known as binary JSON, or BSON. However, MongoDB is a JSON database, as far as developers are concerned.
Explore the MongoDB course syllabus and enhance your skills with us.
Grouping Documents: Collections
Documents are gathered into collections in MongoDB. You can think of a collection as a table if you are familiar with relational databases.
However, MongoDB collections offer much greater flexibility. Documents inside the same collection may have varying fields, and collections do not require a schema.
Every collection has a single MongoDB database linked to it. Use the listCollections command to display which collections are present in a specific database.
Maintaining high availability: Replica Sets
To guarantee high availability, it’s crucial to maintain multiple copies of your data. High availability is ingrained in the design of MongoDB.
When you create a database, MongoDB automatically creates a replica set, which is two or more additional copies of the data. A replica set consists of three or more MongoDB instances that replicate data continuously among themselves to provide redundancy and prevent downtime in the event of planned maintenance or a system failure.
Capability to Manage Abundant Data Increase: Sharding
A modern data platform must be able to use increasingly larger clusters of modest machines to handle vast datasets and extremely rapid queries. The term “sharding” refers to the clever distribution of data among several devices.
How does MongoDB’s sharding function? MongoDB distributes documents inside a collection among the shards in a cluster by sharding data at the collection level. Scale-out architecture that can accommodate even the largest applications is the result.
Increasing Query Performance: Indexes
Indexes help make query execution more efficient. Compound indexes on several fields are among the many indexing algorithms that MongoDB offers.
Well-selected indexes reduce query processing time by allowing queries to scan the index rather than each document in the collection.
Analyzing which searches would benefit from having an index added still needs more effort. Performance Advisor is one tool that can undertake this analysis for you; it evaluates queries and recommends indexes that might enhance query performance.
Quick Data Transfers: Aggregation Pipelines
Data processing pipelines that may be constructed using the adaptable architecture available in MongoDB are known as aggregation pipelines. With its many stages and more than 150 operators and expressions, you may process, transform, and analyze data with any kind of structure on a large scale. The Union stage is a new component that allows for flexible result aggregation from multiple collections.
Our MongoDB training in Chennai at SLA equips you with an understanding of data science fundamentals, Python, and MongoDB skills.
FAQs
Is Python good with MongoDB for the data science process?
Python has an actively maintained library that is updated with new features, security updates, bug fixes, and performance improvements.
How to monitor MongoDB?
With a few utilities and commands, you can check instance status, cluster operations and connections metrics, hardware metrics, and much more to keep an eye on the health and performance of your cluster. Monitoring can assist in identifying and responding to concerns in real-time before they get out of hand.
What is the MongoDB cloud?
Available on all public clouds, MongoDB Atlas is a database-as-a-service variant of MongoDB Enterprise Edition. MongoDB Atlas and other emerging products like Realm, a serverless computing environment for building mobile applications built on MongoDB Atlas, are referred to as MongoDB Cloud.
Suggested Article: Cloud Computing Vs. Data Science
Conclusion
MongoDB can filter, pre-process, and generally design use-case-specific data pipelines using aggregate pipelines, as was previously described. They can be incredibly effective at retrieving enriched and refined data from the pipeline’s output if they are constructed correctly and logic is applied correctly. After building a ‘DataFrame’, it is computationally several times faster than doing the same thing in Python or any other interpretable language. We hope this data science fundamentals with Python and MongoDB article will be helpful for you. Enroll in our data science course in Chennai to get expertise in Python and MongoDB.